Schalten Sie Ihre Erfolgsgeschichte frei: Steigen Sie mit Austrian Gaming Insights auf ein höheres Level
Wir befähigen Sie, Herausforderungen zu meistern und als Sieger hervorzugehen
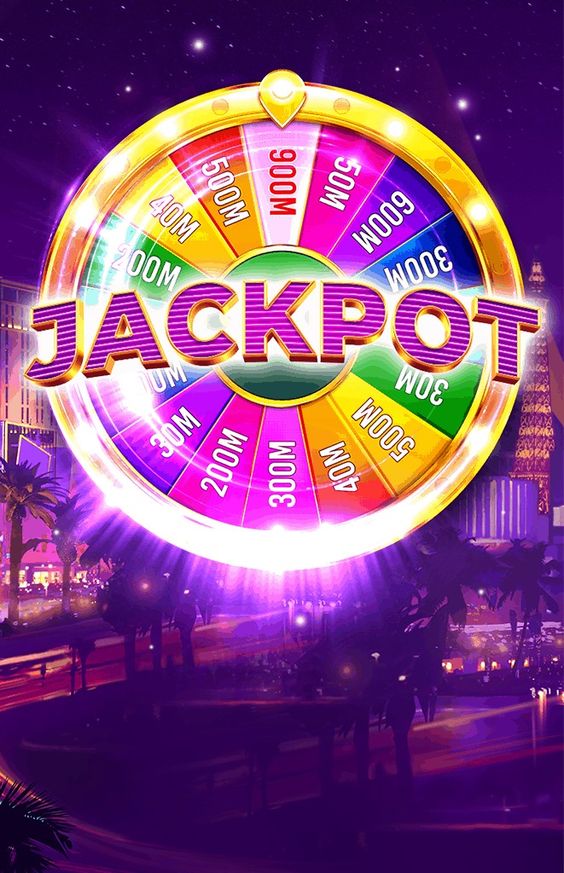
Österreichische Casino-Skandale: Kontroverse Momente der Glücksspielgeschichte
Einführung
Österreichische Casino-Skandale: Kontroverse Momente in der Geschichte des Glücksspiels haben unauslöschliche Spuren im Wett- und Unterhaltungswesen hinterlassen. Diese Skandale haben die Öffentlichkeit in ihren Bann gezogen, von Pokerspielen mit hohen Einsätzen bis hin zu ausgeklügelten Betrugsversuchen. Dieser umfassende Artikel taucht in die Tiefen dieser Kontroversen ein und bietet Einblicke, Enthüllungen und Antworten auf brennende Fragen zu den österreichischen Casinoskandalen. Lassen Sie uns also die Würfel rollen und die Geheimnisse hinter diesen skandalösen Momenten aufdecken.
Österreichische Casinoskandale: Kontroverse Momente in der Geschichte des Glücksspiels
Das Glücksspiel ist seit Jahrhunderten ein fester Bestandteil der österreichischen Kultur. Das Land kann auf eine reiche Geschichte von bestes Online Casino und Wettbüros zurückblicken, aber nur manchmal verlief alles reibungslos. Hier erkunden wir die kontroversesten Momente, die sich negativ auf das Glücksspiel ausgewirkt haben.
Die Rolle von AI und maschinellem Lernen im Online-Casino-Betrieb
In der sich ständig weiterentwickelnden Welt der Online-Casinos spielt die Technologie eine entscheidende Rolle bei der Verbesserung der Benutzererfahrung, der Optimierung der Abläufe und der Gewährleistung eines fairen Spielbetriebs. Künstliche Intelligenz (KI) und maschinelles Lernen (ML) sind zwei hochmoderne Technologien, die die Funktionen von Casino Austria Online Casino erheblich verändert haben. Im Folgenden erfahren Sie, wie diese Innovationen die Welt der Online-Glücksspiele umgestalten.
Verbessertes Engagement der Benutzer
KI- und ML-Algorithmen sind in der Lage, Nutzerdaten, Vorlieben und Verhaltensweisen zu analysieren. Online-Casinos nutzen diese Daten, um ihren Spielern ein personalisiertes Spielerlebnis zu bieten. Durch das Verständnis der individuellen Vorlieben kann die KI Spiele, Boni und Aktionen vorschlagen, die den Spieler eher ansprechen, und so das Engagement und die Bindungsrate erhöhen.
Top Online Casino Seiten in Österreich 2023
In der pulsierenden Welt der Online Casino Österreich nicht hinten. Mit einer Fülle von Anbietern und Spielen, die stetig wachsen, gibt es in diesem Segment immer mehr zu entdecken. Von der Kompatibilität mit Mobilgeräten bis hin zum Einsatz modernster Sicherheitsmaßnahmen, bieten die Online Casino Seiten in Österreich eine unglaubliche Vielfalt an Optionen für Spieler.
Beste Österreichische Online Casinos: Übersicht
Online Casinos in Österreich haben viel zu bieten. Die besten Online Casinos zeichnen sich durch eine beeindruckende Kombination verschiedener Faktoren aus, die von der Spieleauswahl über Boni bis hin zu schnellen Auszahlungen und einer sicheren Spielumgebung reichen.
Die Reise eines Spielautomaten: Von der Idee zum Casino
In der Welt des Glücksspiels und der Unterhaltung haben nur wenige Erfindungen die Vorstellungskraft und die Geldbörsen von Millionen Menschen so sehr beflügelt wie der Spielautomat. Diese ikonischen Geräte sind zu einem Synonym für Kasinos geworden und haben einen faszinierenden Weg von der Idee bis zur Aufstellung im Kasino hinter sich. In diesem Artikel befassen wir uns mit dem komplizierten und faszinierenden Prozess, einen Spielautomaten zum Leben zu erwecken – von der ersten Idee bis zu seinem glamourösen Debüt im Casino.
Konzeption und Brainstorming
Jede großartige Kreation beginnt mit einer einfachen Idee. Im Falle eines Spielautomaten beginnt sie mit einer Vision von Unterhaltung, Spannung und natürlich dem Potenzial für große Gewinne. Spieleentwickler, Designer und Ingenieure setzen sich zusammen, um Konzepte zu entwerfen, die nicht nur die Spieler fesseln, sondern auch den neuesten Trends in der Technologie und den Vorlieben der Spieler gerecht werden.
Initiativen für verantwortungsvolles Spielen in Österreichs Online Casinos
Im Bereich der Online-Unterhaltung und des Online-Glücksspiels hat sich Österreich zu einem wichtigen Akteur entwickelt. Mit seiner reichen Geschichte, seiner lebendigen Kultur und seiner wachsenden Spielergemeinde haben Österreichs Online-Casinos viel Aufmerksamkeit erregt. Mit der Entwicklung der digitalen Glücksspiellandschaft steigt jedoch auch der Bedarf an verantwortungsvollen Glücksspielinitiativen.
Der Aufschwung der Online Casinos in Österreich
Österreich, im Herzen Europas gelegen, war schon immer für seine Leidenschaft für Kunst, Musik und zunehmend auch für Online-Glücksspiele bekannt. Die malerischen Landschaften und charmanten Städte des Landes bieten die perfekte Kulisse für eine florierende Online-Casino-Industrie.